01 Hello World
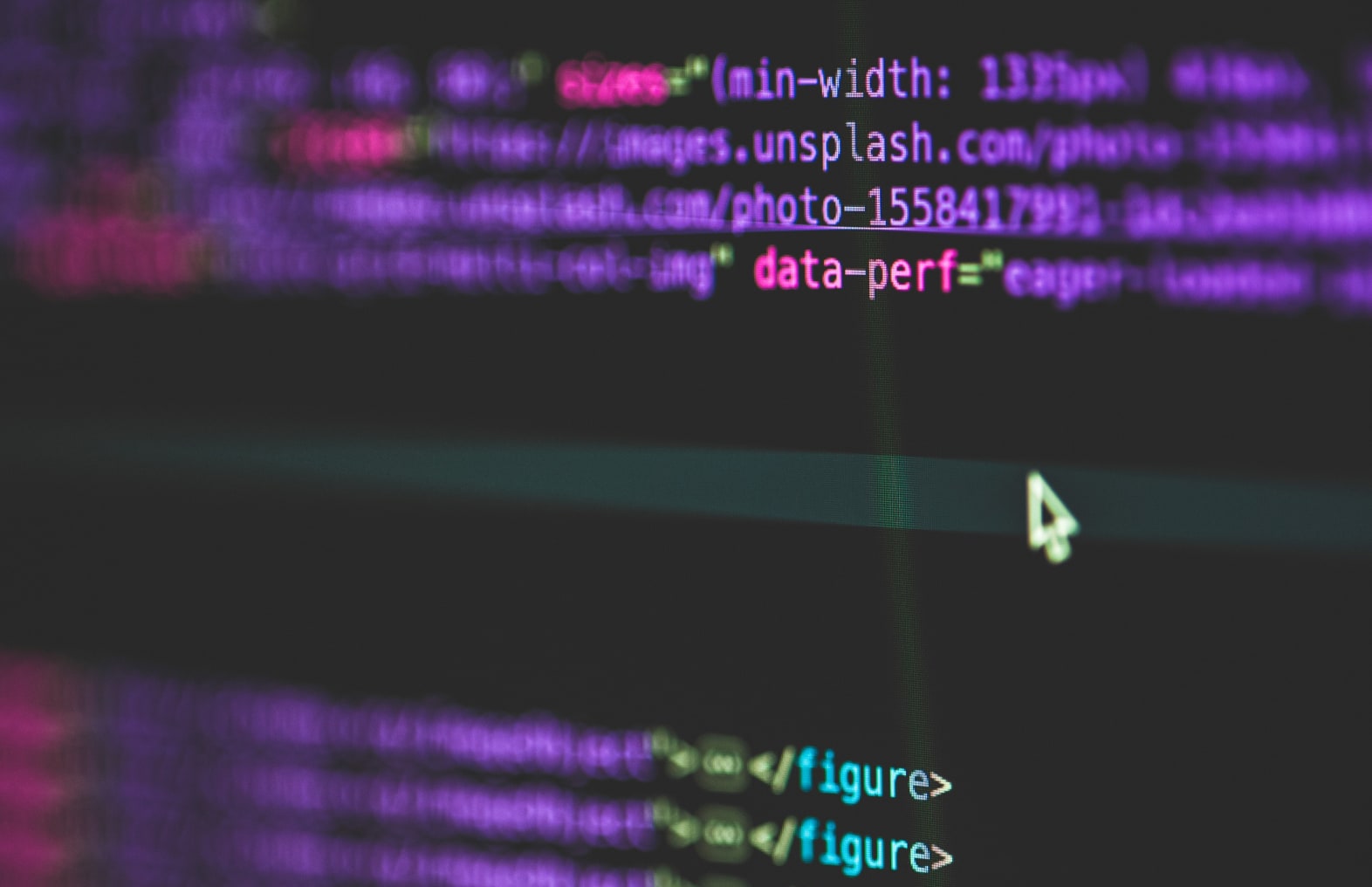
WPF Tutorial 01 – Hello World
- Create a new empty Visual Studio Solution: WPFTutorials
- Add to the solution a new C#, Avalon Application: 01_HelloWorld
- Click Start to see what VS2005 create for you
- Insert in the Grid tag in Window1.xaml a label like this:
<Label>Hello World</Label>
- Click start to test the application
- Add two attributes to the Grid to make the label appear in the middle of the
Window:VerticalAlignment="Center" HorizontalAlignment="Center"
- Start the application and resize the window
- Add a button tag to the Grid:
<Button>Ok</Button>
- Start the application to see how the button hides the label
- Surround the label and button in a new Grid and add row and column
definitions like this:<Grid ShowGridLines="True">
<Grid.RowDefinitions>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
</Grid.ColumnDefinitions>
<Label Grid.Column="0" Grid.Row="0">Hello World</Label>
<Button Grid.Column="1" Grid.Row="1">Ok</Button>
</Grid>
- Start the application to see how the label and button are aligned
- Add a click attribute to the button:
<Button Grid.Column = "1" Grid.Row = "1" Click = "OkButtonClick" >Ok</Button>
- Add an eventhandler to the Window1.xaml.cs file directly under the sample
eventhandler inside the Window1 class:private void OkButtonClick(object sender, RoutedEventArgs e)
{
MessageBox.Show("Hello WPF!");
}
- Start the application and click the button