03 DataBinding
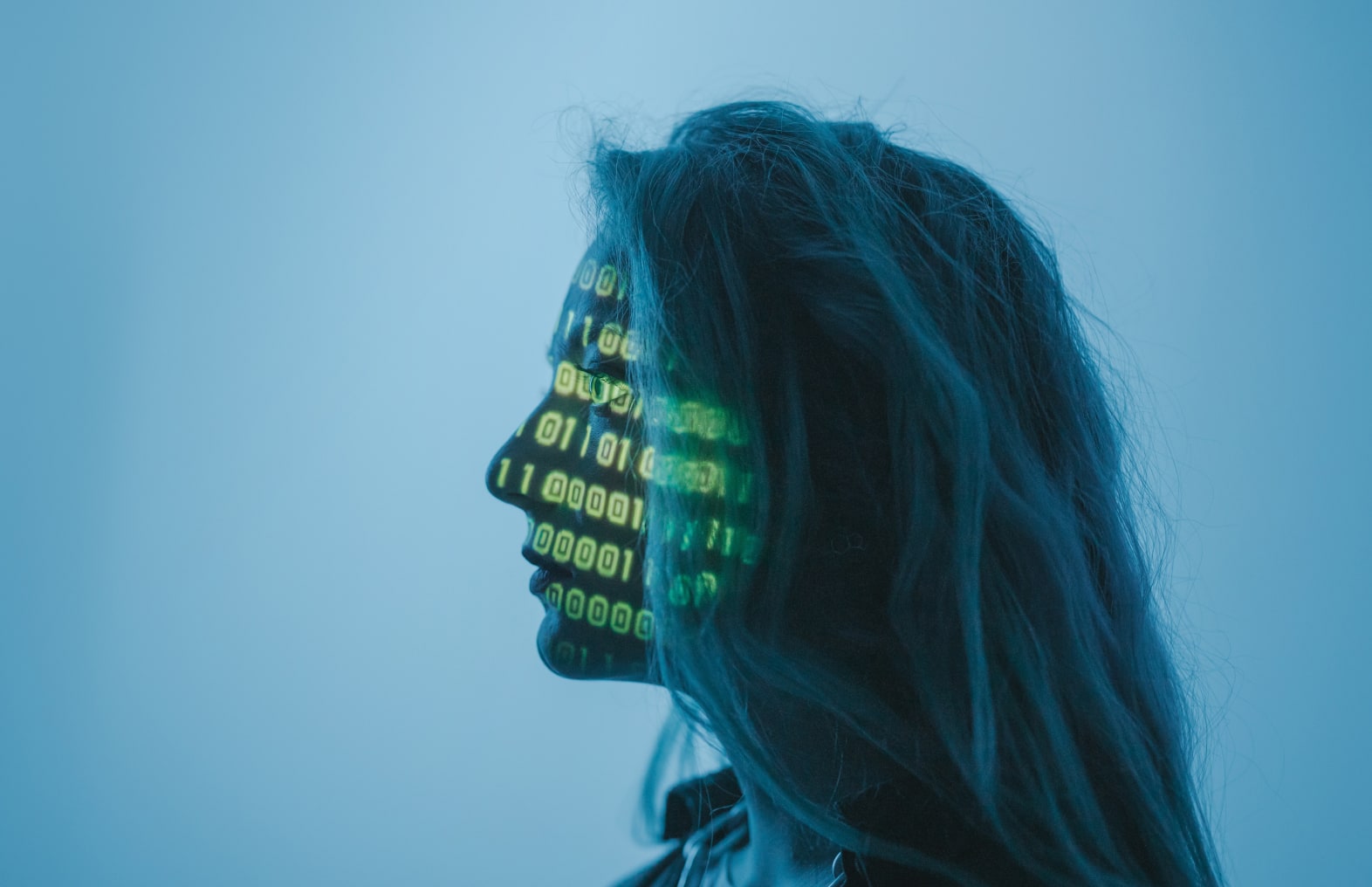
WPF Tutorial 03 – DataBinding
- Open (or create a new empty) Visual Studio Solution: WPFTutorials
- Add to the solution a new C#, Avalon Application: 03_DataBinding
- Add a new class file: Employee.cs that contains the following code:
using System;
using System.Collections.Generic;
using System.Text;
using System.Collections.ObjectModel;
using System.ComponentModel;namespace _03_DataBinding
{
public class Employee : INotifyPropertyChanged
{
private string name;public string Name
{
get { return name; }
set { name = value; OnPropertyChanged("Name"); }
}private DateTime contractDate;
public DateTime ContractDate
{
get { return contractDate; }
set { contractDate = value; OnPropertyChanged("ContractDate"); }
}public Employee()
: this("<New>", DateTime.Now)
{
}public Employee(string name, DateTime contractDate)
{
this.Name = name;
this.ContractDate = contractDate;
}public override string ToString()
{
return Name + " hired since " + ContractDate.ToShortDateString();
}public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(String info)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(info));
}
}
}public class EmployeeList : ObservableCollection<Employee>
{
public EmployeeList()
{
}
}
}
- By implementing INotifyPropertyChanged the Employee class allows the
outside world to get notified of changes to instances of type Employee. The
EmployeeList class uses this interface by inheriting from the generic
ObservableCollection. This construction allows Databinding as we will see. - Add code to the Window1.xaml.cs:
public partial class Window1 : Window
{
public EmployeeList Employees = new EmployeeList();private void InitData()
{
Employees.Add(new Employee("Mr. A", DateTime.Now.AddDays(-1000)));
Employees.Add(new Employee("Mr. B", DateTime.Now.AddDays(-1500)));
Employees.Add(new Employee("Mr. C", DateTime.Now.AddDays(-2500)));
Employees.Add(new Employee("Mr. D", DateTime.Now.AddDays(-750)));
}public Window1()
{
InitData();
this.DataContext = this.Employees;
InitializeComponent();
}
…
- This code creates an Employee list and sets the DataContext. By setting
the DataContext you determine the base upon which the relative binding
expressions (later) will be based. - Add to the Window1.xaml:
<Grid>
<Grid VerticalAlignment="Center" HorizontalAlignment="Center" Width="250"
Height="400">
<Grid.ColumnDefinitions>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="300"/>
<RowDefinition/>
</Grid.RowDefinitions>
<ListBox Grid.Column="0" Grid.Row="0" Name="EmployeeListBox"
ItemsSource="{Binding Mode=OneWay}"
SelectedIndex="0" />
<Button Name ="ChangeContractDatesButton" Click="ChangeContractDatesButtonClick"
Grid.Column="0" Grid.Row="1">Change contract dates</Button>
</Grid>
</Grid>
- This code adds a listbox and a button. The listbox binds to the current
DataContext (our EmployeeList) - Before continueing you should add the following line to the top of
Window1.xaml:<?Mapping XmlNamespace="TutorialDataBinding" ClrNamespace="_03_DataBinding"
?>
- This is to ensure that the namespaces that we use across our xaml files
are equal (we’ll add the same line to the styles xaml) - Then add a Style1.xaml to your project and make it look like this:
<?Mapping XmlNamespace="TutorialDataBinding" ClrNamespace="_03_DataBinding"
?>
<ResourceDictionary >private void ChangeContractDatesButtonClick(object sender,
RoutedEventArgs e)
{
foreach (Employee employee in Employees)
{
employee.ContractDate += TimeSpan.FromDays(-100);
}
}
- This simply loops through the employeelist and changes the
ContractDates so we can see databinding. - Then we have to hookup the Style1.xaml file to the Window1 by
adding this code to Window1.xaml:<Window.Resources>
<ResourceDictionary x:Name ="Style1" Source="Style1.xaml" />
</Window.Resources>
- Run the application.