Added functionality to work with correlated workflows
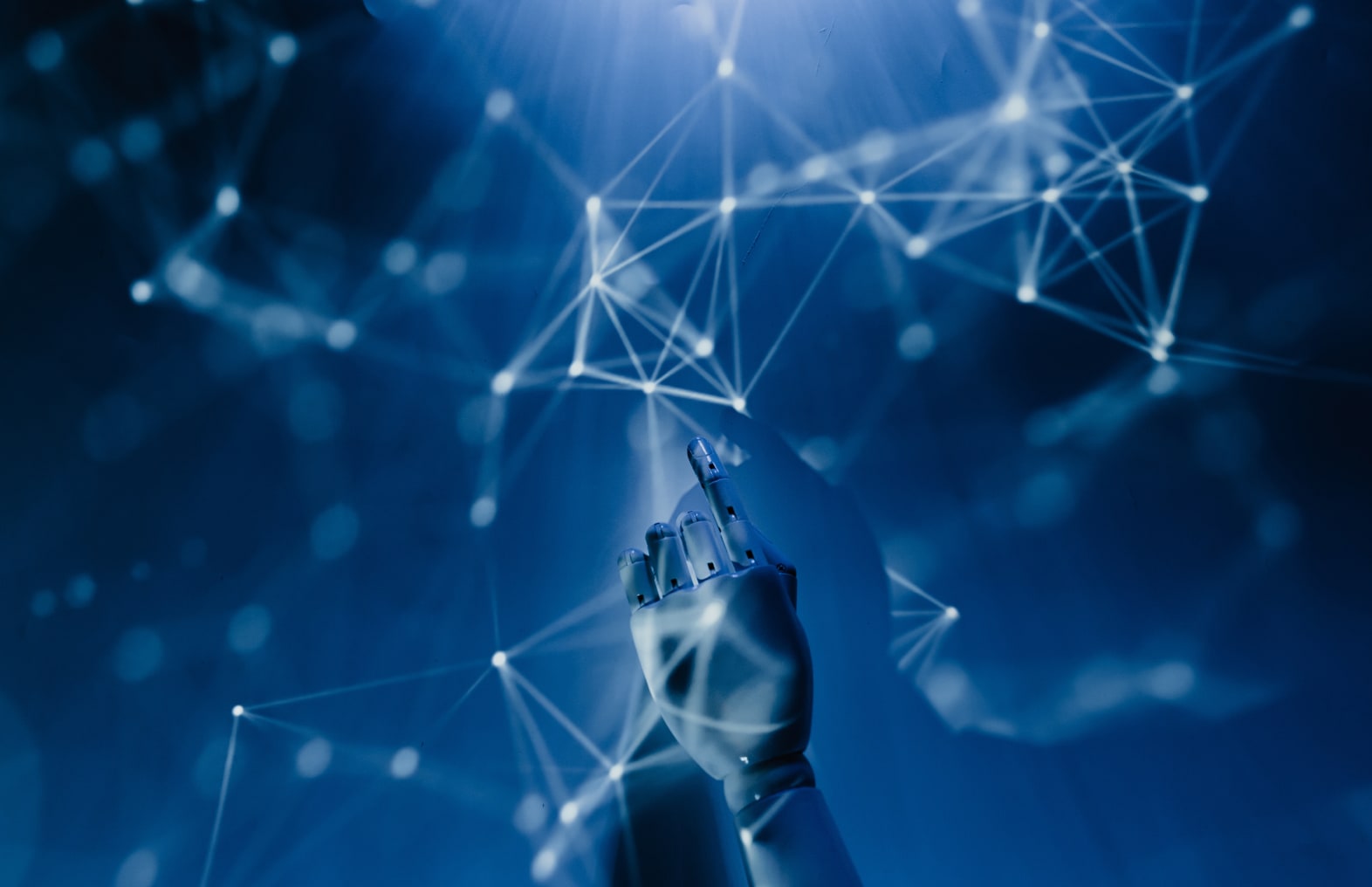
*Moved to: http://fluentbytes.com/added-functionality-to-work-with-correlated-workflows/
This evening I implemented the rudimentary way of doing workflow correlation in the WCFInputActivity
You can now create a workflow that has multiple WCFInputActivities and have the next calls correlated to the same workflow instance. The way it works is based on the use of a custom SOAP header you can add before you call the WCF service implementation.
To give you an idea how it works:
// Instantiate the generated proxy
wcfTest.WCFInputActivity_wcfContractImplClient client = new WCFTestClient.wcfTest.WCFInputActivity_wcfContractImplClient();
using (OperationContextScope scope = new OperationContextScope(client.InnerChannel))
{
Guid WorkflowID =
client.EchoMessageSendToWorkflow("Hello workflow with WCF");
// now do the call again and add a message header to corelate the workflow
MessageHeader<Guid> mhg = new MessageHeader<Guid>(WorkflowID);
MessageHeader untyped = mhg.GetUntypedHeader("WorkflowInstanceID",
"WCFInputActivity");
OperationContext.Current.OutgoingMessageHeaders.Add(untyped);
string msg = client.GetOriginalMessageBackFromWorkflow();
MessageBox.Show(msg);
client.Close();
}
On the server side I now implemented the method to get the instance from the header
Guid workflowInstanceID = OperationContext.Current.IncomingMessageHeaders.GetHeader<Guid>("WorkflowInstanceID", "WCFInputActivity");
return workflowRuntime.GetWorkflow(workflowInstanceID);
For future extension it is now possible to add a custom behavior that injects the custom header based on e.g. a database lookup in your tracking store or some other mechanism to retrieve the workflowID based on input parameters.
During this work I ran into some subtle bugs regarding handling parameter less methods as well, so that is fixed right away. The test workflow I use now looks as follows:
After a few more little things I will create a 0.5 release, so you don’t have to download the changesets separately anymore. Hope I have enough time to do this before the end of the week.
Go to codeplex and get the latest change sets I just checked in. (http://www.codeplex.com/WCFWorkflow/SourceControl/DownloadSourceCode.aspx?changeSetId=286)
Follow my new blog on http://fluentbytes.com