Back to basics (or rather: What they all will be laughing at in a century) Part II
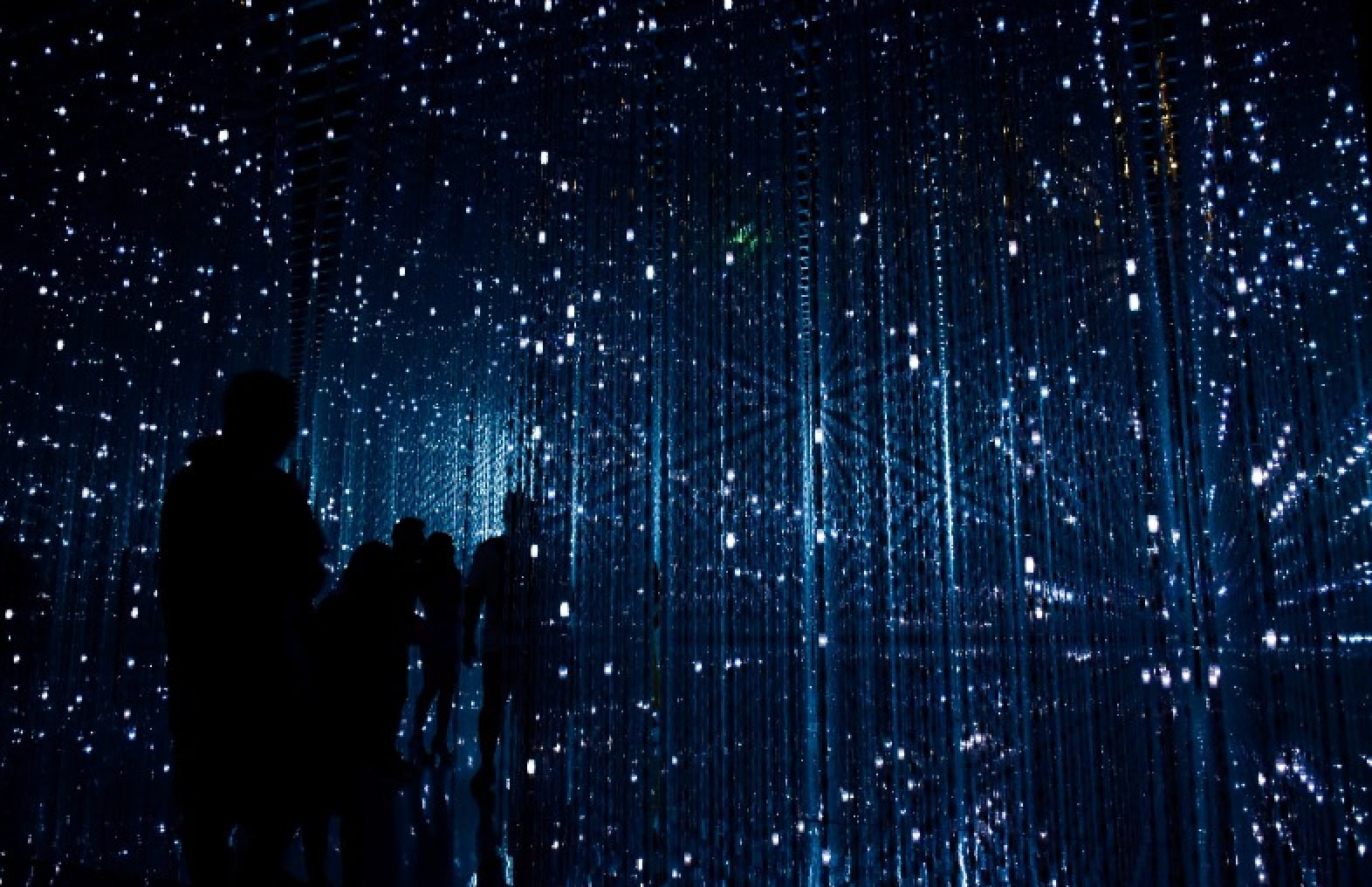
One thing that has amazed me since I learned Java and C# is the way history keeps interfering with ‘modern’ languages. The first thing you learn when picking up a new language is the famous Hello World. The ‘Hello World’ – code in Java and C# is very similar:
Java:
|
C#
|
public class Hello
{
public static void main(String[] args) {
System.out.println(“Hello, world!”); } } |
class Hello
{ public static void Main(string[] args) { System.Console.WriteLine(“Hello, world!”); } } |
In both sources there is a class that contains a static method called Main. This is the entry point of the application. In other words: When we ‘start’ the application the Main method is started. Starting the application is different in Java and C#:
Java:
|
C#
|
c:>java.exe Hello.class
Hello, world!
c:>_
|
c:>Hello.exe
Hello, world!
c:>_
|
As you can see, to start a Java program you instruct the Java environment to run a class whereas .NET applications have a Main marked (by the compiler) that will be started by the .NET environment.
Now, who started taking Main for granted? Wouldn’t it be more sensible, logical, Object Oriented to have a constructor as entry point? So hereby I suggest the following brand new language E# (sorry couldn’t resist):
E# Hello World
|
E# Execution
|
public class Hello
{
public void Hello(string[] args)
{
System.Console.WriteLine(“Hello, World!”);
}
}
|
c:>MyApplication.exe Hello
Hello, World!
c:>_
|
Note that by passing the name of the class I am now able to choose what class will be started (a bit like Java) and a more Object Oriented approach by instantiating an object (The Program).