Bye PCL, Hello .NET Standard
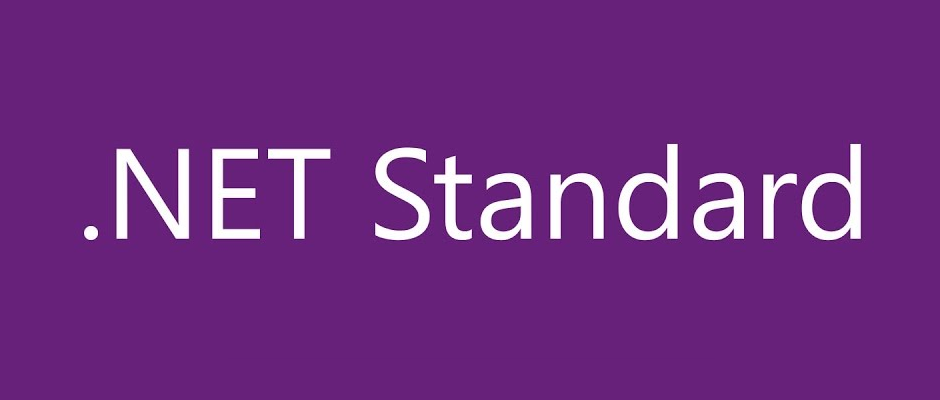
When building a cross-platform application it is really important to be able to share code between the different platforms. This is obviously one of the reasons we pick Xamarin for our mobile development.
Back in the days Xamarin didn’t have a lot of code sharing options as they do now. Actually, the common way of sharing code was using Shared Projects.
Shared Projects made it possible for us developers to share code across different platforms. However, personally, I think the way Shared Projects work isn’t that pretty and it is also hard to maintain.
Shared Projects let you write common code that is referenced by a number of different application projects.
The code in a shared project is compiled as part of each referencing project. Compiler directives are there to help implement platform-specific functionality in the shared code base. The biggest disadvantage of this approach, in my opinion, is the lack of maintainability combined with not actually having a shared output library.
So, how does this thing work? You start out with creating a project that has shared code in it and add the files as link into the project. If you would like to do some platform specific code you can do the following:
#if __Android__ {code for android} #endif
Xamarin has a great example of how this works implemented in their Tasky application. The following illustration shows the architecture of an application using Shared Projects.
After reading this example I actually have to take two showers to wash off the dirty feeling. All jokes aside, this approach helped us a lot back in the days, but nowadays we really want a different approach that allows us to share a library (dll) across platforms.
Portable Class Library
That’s where PCL’s (Portable Class Libraries) come into the picture. This was Microsoft’s answer on the whole code sharing problems. With a PCL it was possible to create a shared library which targeted specific platforms, for example Xamarin.iOS.
The Portable Class Library worked great, but Microsoft was feeling the need for a standard. The .NET framework was forked quite a lot over the years which caused a massive problem for developers since they weren’t able to target one unified class library. So the current architecture looks like the image below.
As you can see there are three flavors of .NET which requires you to know three different base class libraries in order to write cross-platform code. Luckily Microsoft has a great solution for this: .NET Standard.
As you can see .NET standard has only one base class library. For developers this means that they only have to know a single base class library. Libraries that are targeting .NET Standard run on all .NET platforms.
Compared to PCL
So how does this exactly differ from a PCL again? With PCL’s you select which platforms you would like to target and the tooling presents you with an API set that you’re able to use. Even though the tooling helps you out with selecting the right API’s it still means you have to know each base class library.
With .NET Standard every API is supported on every platform. In addition, the .NET Standard is very predictable because each higher version means more API coverage.
So, what API’s are available in .NET Standard?
At the start of the development of .NET Standard Microsoft decided they would start with all the API’s covered in .NET Framework and Xamarin.
Microsoft uses a particular process where they group API’s in two different categories; required and optional.
Required means that Microsoft thinks these specific API’s are what all platforms need and are able to be implemented for cross-platform usage.
Optional on the other hand means that an API is platform-specific or is part of legacy technology.
The current .NET Standard API Coverage looks like this. Microsoft is still in development which means more API coverage will be added in the future.
I really hope that reading this cleared some stuff up about the new .NET Standard. To be honest this is really just the tip of the iceberg. If you are interested in learning more about .NET Standard, check out: https://blogs.msdn.microsoft.com/dotnet/2016/09/26/introducing-net-standard/