Creating a WCF 4 dynamic locator service part 2! Discovery extensions
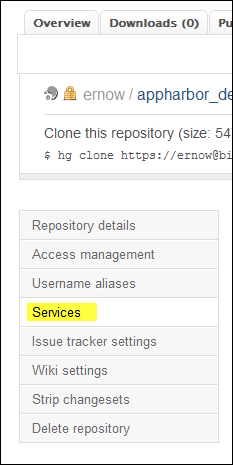
Welcome back again! It has been a while since my last post. Last time I blogged about creating a locator service with WCF 4 discovery features. That article now resides here https://accblogs.infosupport.com/author/chrisb/. It will be shown right below this article. In that post I described how to use WCF 4 discovery to create a locator service that other services will use to announce themselves and a client can use to discover a address and binding based on a given contract.
The only problem with that solution was that discovery doesn’t provide us with Binding information of a service. It will only provide us with an address and the xml qualified name of a contract. To get the binding information I used dynamic meta data resolving, but that meant that all the services that want to be discoverable also needed to provide meta data via http get.
In this article I wan to explore another feature of discovery: Extensions. The discovery mechanism allows us to add extra data from endpoints to the discovery information. You can do this via the EndPointDiscoveryBehavior. This is an endpoint behavior which you can apply in your config file like below:
The endpointDiscovery behavior defines an extensions tag. Within this tag you are free to apply whatever extra information you want! Pretty cool right? What would be even cooler? If we could add this extra information dynamically, via code. This can be done by defining a new behavior. We could define a service behavior that in code applies this EndPointDiscovery behavior and fills the extension collection of this discovery behavior with runtime information. What kind of information you might wonder? Well how about the binding information! We can use this to place the assembly qualified typename of the binding that an endpoint is using, we can then use this information on the client side to dynamically instantiate the correct binding! First let’s define a service behavior that will add this binding information to the EndpointDiscoveryBehavior’s extensions collection. The code:
1: [AttributeUsage(AttributeTargets.Class, Inherited = false, AllowMultiple = false)]
2: public class DiscoveryBindingAttribute : Attribute,IServiceBehavior
3: {
4: public void AddBindingParameters(ServiceDescription serviceDescription, System.ServiceModel.ServiceHostBase serviceHostBase, System.Collections.ObjectModel.Collection<ServiceEndpoint> endpoints, System.ServiceModel.Channels.BindingParameterCollection bindingParameters)
5: {
6:
7: }
8: public void ApplyDispatchBehavior(ServiceDescription serviceDescription, System.ServiceModel.ServiceHostBase serviceHostBase)
9: {
10: foreach (var endpoint in serviceHostBase.Description.Endpoints)
11: {
12: EndpointDiscoveryBehavior discoBehavior= new EndpointDiscoveryBehavior();
13: discoBehavior.Extensions.Add(new System.Xml.Linq.XElement("Binding",endpoint.Binding.GetType().AssemblyQualifiedName));
14: endpoint.Behaviors.Add(discoBehavior);
15: }
16: }
17: public void Validate(ServiceDescription serviceDescription, System.ServiceModel.ServiceHostBase serviceHostBase)
18: {
19:
20: }
21: }
The interesting part begins on line 8. Here we iterate over all the endpoints in the service this behavior is applied to. We instantiate a new EndPointDiscoveryBehavior and we add a new XElement to its extensions collection. This XElement represent the custom information we saw earlier in our config file, this is the way to fill this information in code. This is all there is to it! to use this behavior we must apply it to a discoverable service. Like our Calculator service, and our Locator service, both will be discoverable, the Calculator via announcements, the Locator service via UDP.
Here is the Calculator Service code:
1: using System;
2: using System.Collections.Generic;
3: using System.Linq;
4: using System.Runtime.Serialization;
5: using System.ServiceModel;
6: using System.ServiceModel.Web;
7: using System.Text;
8: using Contracts;
9:
10: namespace CalculatorService
11: {
12: [DiscoveryExtensions.DiscoveryBinding]
13: public class CalculatorService : ICalculator,ICalculatorMaintenance
14: {
15:
16: public int Add(int i, int i2)
17: {
18: return i + i2; ;
19: }
20:
21: public bool StatusOk()
22: {
23: //perform checks, everything ok
24: return true;
25: }
26: }
27: }
Now we have the extra binding information exposed via discovery, so we don’t need meta data exchange anymore, here is the updated web.config file without meta data exposure!
Here we can see two endpoints. And we can see that this service announces itself to an endpoint. That endpoint is an endpoint on our locator service. When the locator service receives an announcement, it will store the data that comes with it. This data now included our extra binding information that we added in our extensions!
The locator service now returns two types of info when queried for an contract. It will send back the address of a service that implements that contract, and it will send back the assembly qualified typestring of the binding that that service is using. Here is the code of the locator service:
1: [DiscoveryBindingAttribute]
2: [ServiceBehavior(ConcurrencyMode=ConcurrencyMode.Multiple,InstanceContextMode=InstanceContextMode.Single,IncludeExceptionDetailInFaults=true)]
3: public class LocatorService :AnnouncementService, IServiceLocator
4: {
5: private System.Collections.Concurrent.ConcurrentDictionary<XmlQualifiedName, EndpointDiscoveryMetadata> _services= new System.Collections.Concurrent.ConcurrentDictionary<XmlQualifiedName,EndpointDiscoveryMetadata>();
6: public LocatorService()
7: {
8: this.OnlineAnnouncementReceived += new EventHandler<AnnouncementEventArgs>(anService_OnlineAnnouncementReceived);
9: this.OfflineAnnouncementReceived += new EventHandler<AnnouncementEventArgs>(anService_OfflineAnnouncementReceived);
10: }
11:
12: void anService_OfflineAnnouncementReceived(object sender, AnnouncementEventArgs e)
13: {
14: EndpointDiscoveryMetadata ed;
15: _services.TryRemove(e.EndpointDiscoveryMetadata.ContractTypeNames[0], out ed);
16: }
17:
18: void anService_OnlineAnnouncementReceived(object sender, AnnouncementEventArgs e)
19: {
20: // Note this will be called for every endpoint and every endpoint will have 1 contract, a service has more contracts
21: if (!_services.ContainsKey(e.EndpointDiscoveryMetadata.ContractTypeNames[0]))
22: {
23: _services[e.EndpointDiscoveryMetadata.ContractTypeNames[0]]= e.EndpointDiscoveryMetadata;
24: }
25: }
26:
27: public ServiceDiscoData Discover(XmlQualifiedName contractname)
28: {
29: ServiceDiscoData data = null;
30: EndpointDiscoveryMetadata meta=_services[contractname];
31: if (meta!=null)
32: {
33: data = new ServiceDiscoData() { Adres = meta.Address.ToString(), BindingTypeName = meta.Extensions[0].Value };
34: }
35: return data;
36: }
37: }
The cool stuff begins on line 27. Here the service will retrieve its stored announcements information by using the contract name of a service. From this info he will get the address and the binding typestring, it will then wrap this in a response and send it back to the client.
As in my earlier post, the client is still a dll, this dll will pack a class that will handle the queries to the locator service for us. All a client application needs to do, is to reference our dll and use the DynamicChannelFactory class to create a runtime proxy, all the client application needs to pass is a generic type parameter for the contract type. Our dll will discover the locator service, query it for the information it needs, and then use the WCF ChannelFactory class to instantiate a proxy. To the ChannelFactory class it will pass the binding, and the address. Here is the code of the DynamicChannelFactory class.
1: public static class DynamicChannelFactory
2: {
3: public const int MAXRETRIES = 1;
4: // this will only happen once during te application,or more often when something goes wrong
5: private static ServiceLocator.IServiceLocator _locatorProxy = CreateLocatorProxy();
6:
7: public static TContract CreateChannel<TContract>()
8: {
9: var result = GetDiscoData(typeof(TContract));
10: if (result != null)
11: {
12: return ChannelFactory<TContract>.CreateChannel(result.Item2, new EndpointAddress(result.Item1));
13: }
14: return default(TContract);
15:
16: }
17:
18:
19: public static Tuple<string, Binding> GetDiscoData(Type contract)
20: {
21: Binding b = null;
22: bool error = false;
23: int nrRetries = 0;
24: ServiceDiscoData data = null;
25: ContractDescription cd = ContractDescription.GetContract(contract);
26: do
27: {
28: try
29: {
30:
31: data = _locatorProxy.Discover(new XmlQualifiedName(cd.Name, cd.Namespace));
32: error = false;
33: }
34: catch (FaultException)
35: {
36: // service was not found
37: return null;
38: }
39: catch (CommunicationException)
40: {
41: // something infra structural went wrong, maybe the locator service moved? Lets discover it again and try again
42: CreateLocatorProxy();
43: error = true;
44: nrRetries++;
45: }
46:
47: } while (error && nrRetries < MAXRETRIES);
48:
49: if (data != null)
50: {
51: b = Activator.CreateInstance(Type.GetType(data.BindingTypeName)) as Binding;
52: return new Tuple<string, Binding>(data.Adres, b); ;
53: }
54: else
55: {
56: return null;
57: }
58:
59: }
60:
61: /// <summary>
62: /// Finding via udp is still relatively slow, this will happen only the first time, if there is a well known address of this service
63: /// its better to use that instead of locating it dynamically, its also better to define a well known binding for its endpoint.
64: /// </summary>
65: /// <returns></returns>
66: private static ServiceLocator.IServiceLocator CreateLocatorProxy()
67: {
68: Type locatorContract = typeof(ServiceLocator.IServiceLocator);
69: DiscoveryClient dc = new DiscoveryClient(new UdpDiscoveryEndpoint());
70: FindCriteria criteria = new FindCriteria(locatorContract);
71: //dont forget to set the max results to one, this is a huge performance improvement
72: criteria.MaxResults = 1;
73: FindResponse response = dc.Find(criteria);
74: if (response.Endpoints.Count > 0)
75: {
76: var metaExchangeEndpointData = response.Endpoints[0];
77:
78: // The Locator service only has one endpoint which support locating
79: return new ServiceLocator.ServiceLocatorClient((Binding)Activator.CreateInstance(Type.GetType(metaExchangeEndpointData.Extensions[0].Value)), metaExchangeEndpointData.Address);
80:
81: }
82: return null;
83: }
84: }
Line 66 is first up. This method will create a locator proxy for us so that we can ask it for service addresses and bindings. The factory will create a locator proxy as soon as it is initialized. it will try to discover the locator service by using udp. Because we also annotated our locator service with our cool new extension attribute, a response by the locator service will also include our XElement with binding information. This information is used to instantiate a proxy that was earlier generated with svcUtil. No ChannelFactory here.
Later on the GetDiscoData of our DynamicChannelfactory will use the locator proxy to query for service data, You can see this method from line 19. It will try to query, if it fails it will try to generate a new locator proxy and then query again. This method returns a tuple of an adress and a binding. The CreateChannel method is the method that a client should call to dynamically generate a proxy, this will use the WCF ChannelFactory to generate a proxy, by first querying our Locator service for address and binding data.
That’s it! Pretty cool stuff! Now we only need to know contracts and the rest will be searched for by runtime! Ideal for mocking etc. By using the extension mechanism of Discovery we have now one less method call for every query, and the services don’t have to expose metadata anymore!