Finding unused code
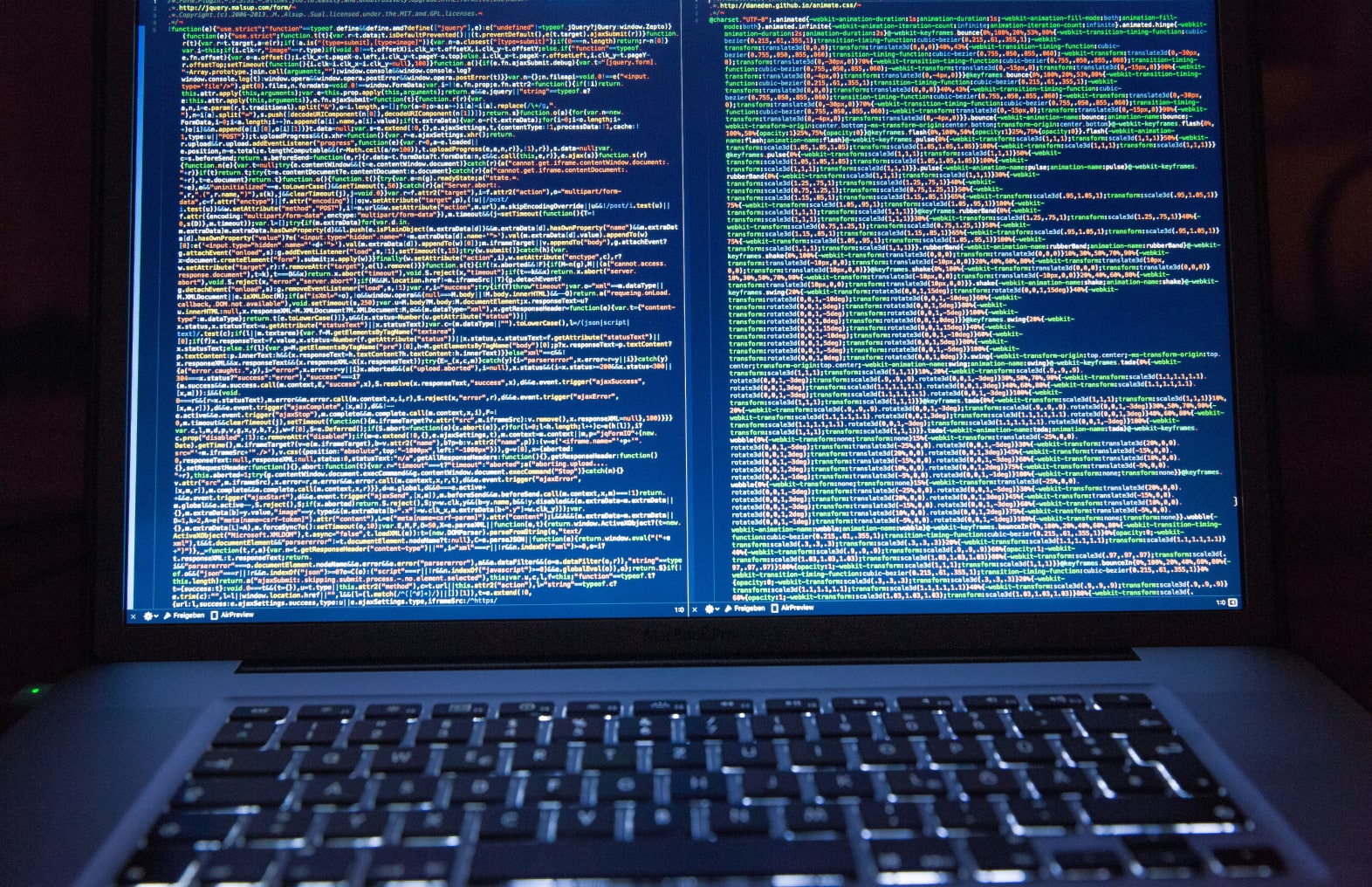
Finding code that can be thrown away is a highlight of my day. Deleting code means it no longer has to be compiled, maintained or tested. No one has to see the code and wonder "hmm, what's this doing here?".
Especially on big projects with shifting insights in features or architecture and design, old code tends to be left behind. To determine if code is really unused is hard and may take a lot of manual inspection. Fortunately, the Eclipse Core Tools contains a utility called "Find unused members". It tries to determine if code is no longer used.
Download and install the Core Tools plug-in. As an example, consider this code:
package com.infosupport.demo.unused;
import java.io.Serializable;
public class SimpleTalker implements Talker, Serializable {
private static final long serialVersionUID = 1L;
public String sayHello(String message) {
return "Hello " + message;
}
public String sayGoodBye(String message) {
return "Goodbye " + message;
}
public String say(String message) {
return message;
}
public static void main(String[] args) {
System.out.println(new SimpleTalker().sayHello(args[0]));
}
}
package com.infosupport.demo.unused;
public interface Talker {
public String say(String message);
}
(Note that you can copy paste this code to Eclipse and it will generate an example project for you). If you right click on the Java Project you will get a new option:
When you run it, you get the following results:
Note that not all the unused elements are actually unused. The main method and serialVersionUID are special in Java and may be invoked by the VM. Code that is invoked through reflection is not detected. If code that calls this code is not in your workspace (or does not use a project reference to link to the code), it is not detected. Conversely, if you call code only from your unit tests, this tool will not report it because it is still used in the tests. Remember after removing code to re-run the tool, as now more code may have become unused because it was only called from unused code.
Still a lot of gotchas, but nevertheless a great first scanning tool to get rid of that old junk!