HOWTO: bulk export / import MOM management packs
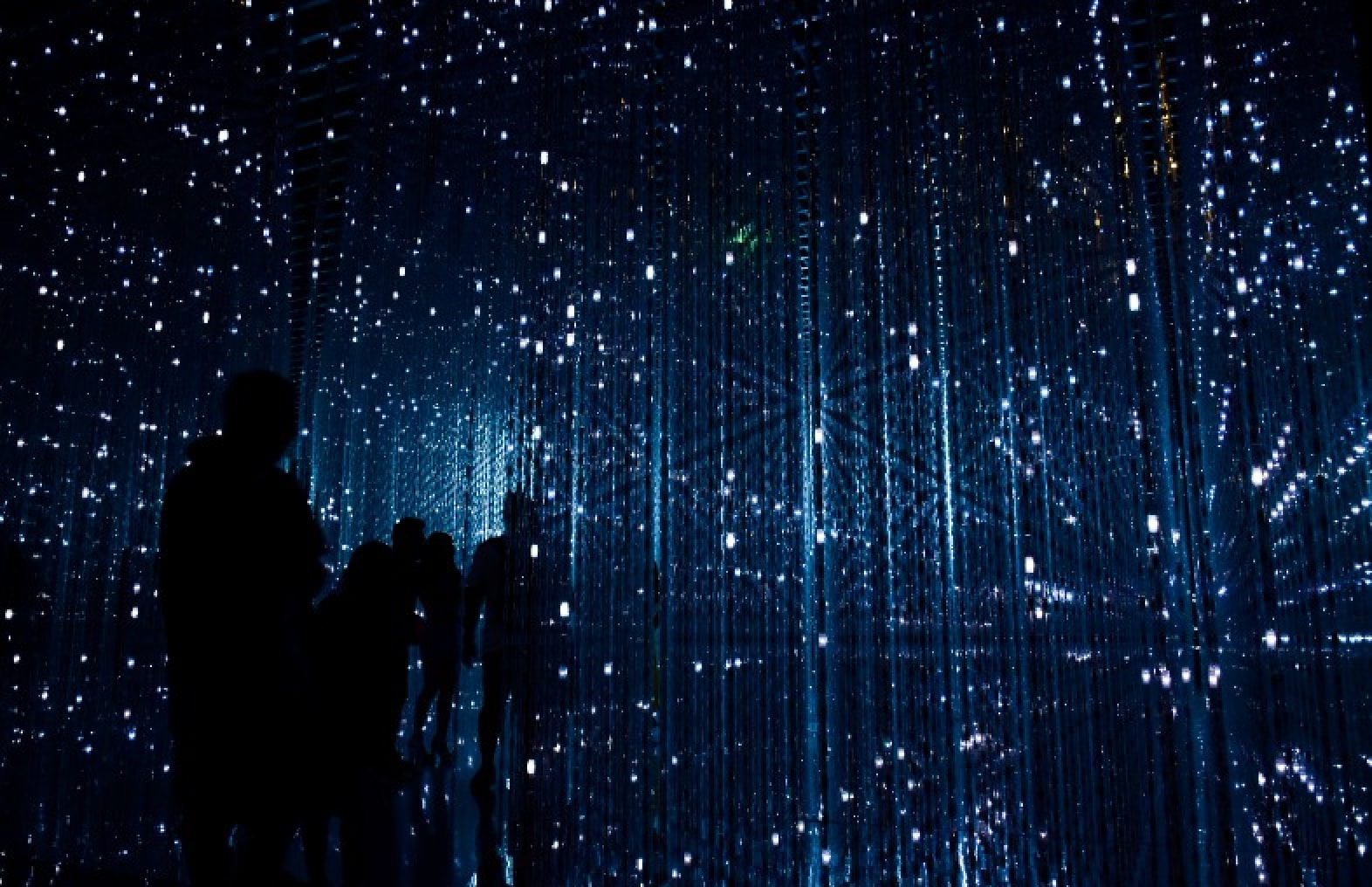
Hi,
As a MOM system administrator / consultant I have visited many customers that don't backup the MOM MPs. The say "why? I'm making a SQL onepoint backups , this should do it….” Personally I'm not a big pro on restoring the complete onepoint db if you lose or miss-change a MP. So the mom SDK has some tools to export / import MPs from the command line. The only problem is that this tool only processes 1 rule group at the time. I've written a c# program that solves this problem. This is a simple way to export (backup) your MPs. I use the tool for one reason more: I'm using the MOM to MOM product connector to forward alerts from a co hosting MOM locations to our main MOM location. This requires you to have the same MPs on both sites. My tool has a Import feature as well. So you can make a co-site export and import to the main site in one step.
So if you want to make only a export (for backup resons) you make the source and destination server parameters the same. The tool then only exports the MPs to the given directory. If you fill in a different destination server the tool will imports the exported MPs one by one on the specified server. The tool also export / imports all the RULES , VIEWS , TASKS.
Command line usage:
ExportImportMP.exe <source mom server> <dest mom server> <dest path>
requirements:
– Dot Net 1.1 framework installed
– MOM SDK installed
– must exists:Program FilesMicrosoft Operations Manager 2005ManagementModuleUtil.exe
– execute on the MOM server.
I will share the code. This is a quick programming project , so do not except to much:
(You can download the project from the download section on this blog server)
It is build of 3 parts.
Part one reads out the command line arguments and is creating a MOM management object.
System.Diagnostics.Process proc = new System.Diagnostics.Process();
string arguments;
string SourceSrv;
string DestSrv;
string DestDir;
if (args.Length != 3)
{
Console.WriteLine("Error invalid arguments");
Console.WriteLine("VERSION : 0.9");
Console.WriteLine("Michel Kamp. 2006");
Console.WriteLine("Usage: ExportImportMP <source mom server> <dest mom server> <dest path>");
Console.WriteLine("Description: Export and import MOM MPs with the ManagementModuleUtil.exe");
Console.WriteLine(@"Description: besure C:Program FilesMicrosoft Operations Manager 2005ManagementModuleUtil.exe exsists");
Console.WriteLine("HINT: If the Source and destionation is the same then no import will be done. This is usefull for making an backup. ");
Environment.Exit(-1);
}
SourceSrv = args[0].ToString();
DestSrv = args[1].ToString();
DestDir = args[2].ToString();
Console.WriteLine("ExportImportMP " + SourceSrv + " " + DestSrv + " " +DestDir );
Administration admin = Administration.GetAdministrationObject();
RuleGroupsCollection rgs = admin.GetRuleGroups();
Part 2 does the export
foreach (RuleGroup rg in rgs)
{
Console.WriteLine("Export RULES: " + rg.Name);
Console.WriteLine("DestDir: " + DestDir);
arguments = " -O " + SourceSrv + " {" + rg.Id.ToString().ToUpper() + "} " + """ + DestDir + @"" + rg.Name + @".AKM" + """ + " -W";
proc.EnableRaisingEvents=true;
proc.StartInfo.FileName=@"C:Program FilesMicrosoft Operations Manager 2005ManagementModuleUtil.exe" ;
proc.StartInfo.Arguments=arguments;
Console.WriteLine("Processing :" + proc.StartInfo.FileName + " " + proc.StartInfo.Arguments);
proc.Start();
…….
Part 3 does the import
if ( SourceSrv != DestSrv )
{
Console.WriteLine("IMPORT: " + rg.Name);
arguments = " -I " + DestSrv + " "" + DestDir + @"" + rg.Name + ".AKM" + "" -F";
proc.EnableRaisingEvents=true;
proc.StartInfo.FileName=@"C:Program FilesMicrosoft Operations Manager 2005ManagementModuleUtil.exe" ;
proc.StartInfo.Arguments=arguments;
Console.WriteLine("Processing :" + proc.StartInfo.FileName + " " + proc.StartInfo.Arguments);
proc.Start();
proc.WaitForExit();
}
Hope you can use it. If not please let me know why and what’s missing.
You can download the project from the download section on this blog server
Michel Kamp