PDC day 2, Linq: The future of data intensive programming
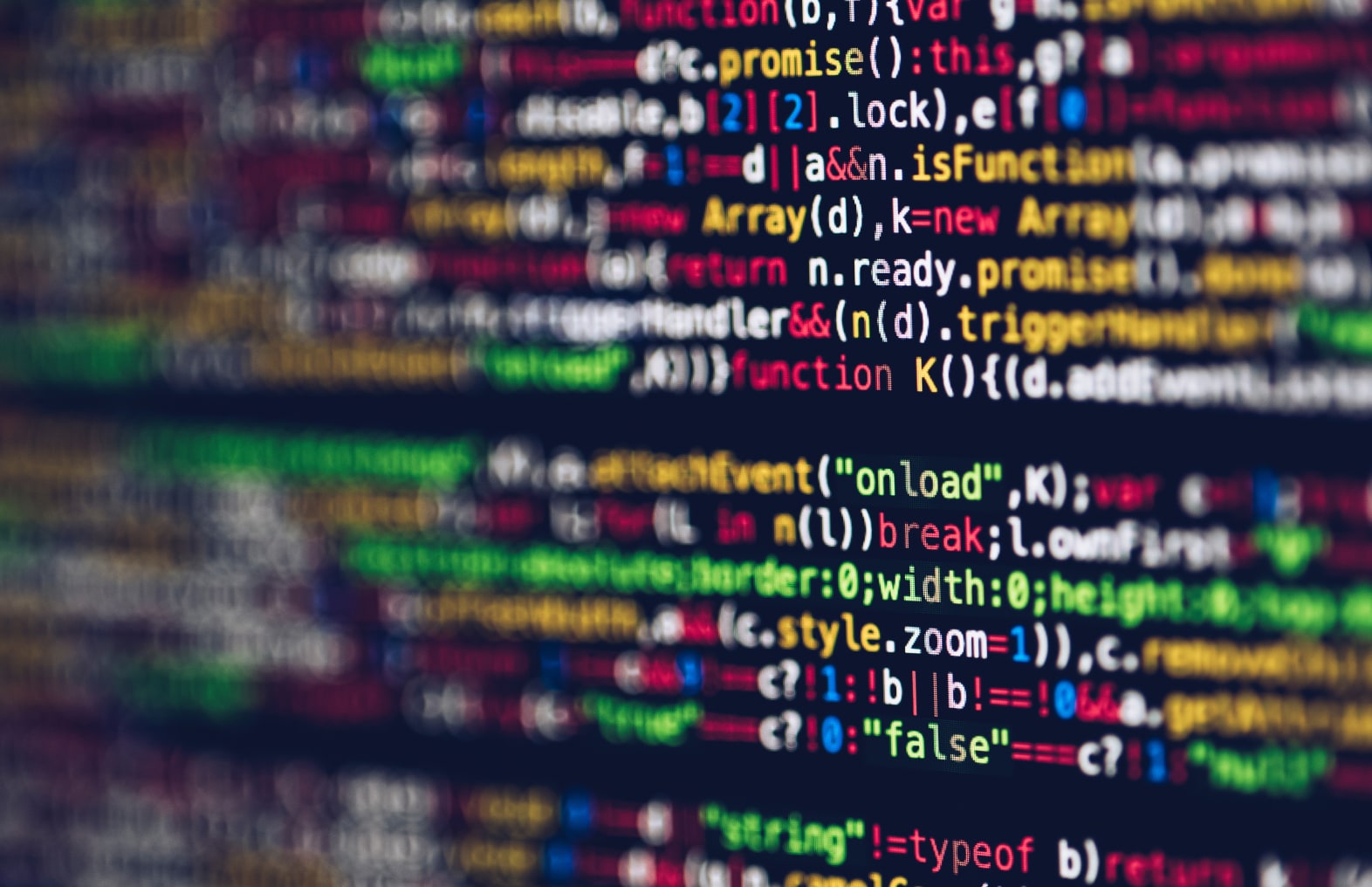
At the PDC, they shown some cool new ways to interact with data from .NET. As you know, there's lots of types of data. You could have DataSets, Object graphs, Xml etc. Furthermore, things become harder in a Service Oriented environment where al these types of data come together.
Often enough you get some data from an external webservice in the form of objects, and a DataSet from a database that you own in your code. The challenge is then to bring that data together and transform it in the correct way for your application.
Furthermore, when using these forms of data, you have different paradigms to adhere to. Xml needs XPath / XSL-T, DataSets use SQL and DataViews and for object graph you just program a bunch of loops and stuff.
Earlier on I had some discussions with some of my collegues at InfoSupport about object-relational mapping. We then came tto the conclusion that we needed a way to do 'client-side' queries, joins etc for datamanipulation and that this should be possible from and to all types of data.
Microsoft has recognized these problems too and came up with Linq (Language Integrated Query) as a framework to do these types of data manipulation. In this framework all types of data can be supported, for instance DLinq as a plug-in for relational data and XLinq as a plugin for Xml.
The really cool thing though is that the whole thing is integrated into the language (currently only C#) and thereby offers things like statement completion (Intellisense) and compiletime checks on your data manipulation.
Compare the following examples:
Today one would do this:
XmlDocument doc = new XmlDocument();
XmlElement contacts = doc.CreateElement("contacts");
foreach (Customer c in customers)
if (c.Country == "USA") {
XmlElement e = doc.CreateElement("contact");
XmlElement name = doc.CreateElement("name");
name.InnerText = c.CompanyName;
e.AppendChild(name);
XmlElement phone = doc.CreateElement("phone");
phone.InnerText = c.Phone;
e.AppendChild(phone);
contacts.AppendChild(e);
}
doc.AppendChild(contacts);
XElement contacts = new XElement("contacts",
from c in customers
where c.Country == "USA"
select new XElement("contact",
new XElement("name", c.CompanyName),
new XElement("phone", c.Phone)
)
);
So this is really cool, and I hope to see this as a finished product soon.. though it wont be available until C#3.0. On the upside, it will run on the whidbey CLR, so that we can already play with the CTP bits today.
[Update: changed sample]