Running JavaFX from Eclipse
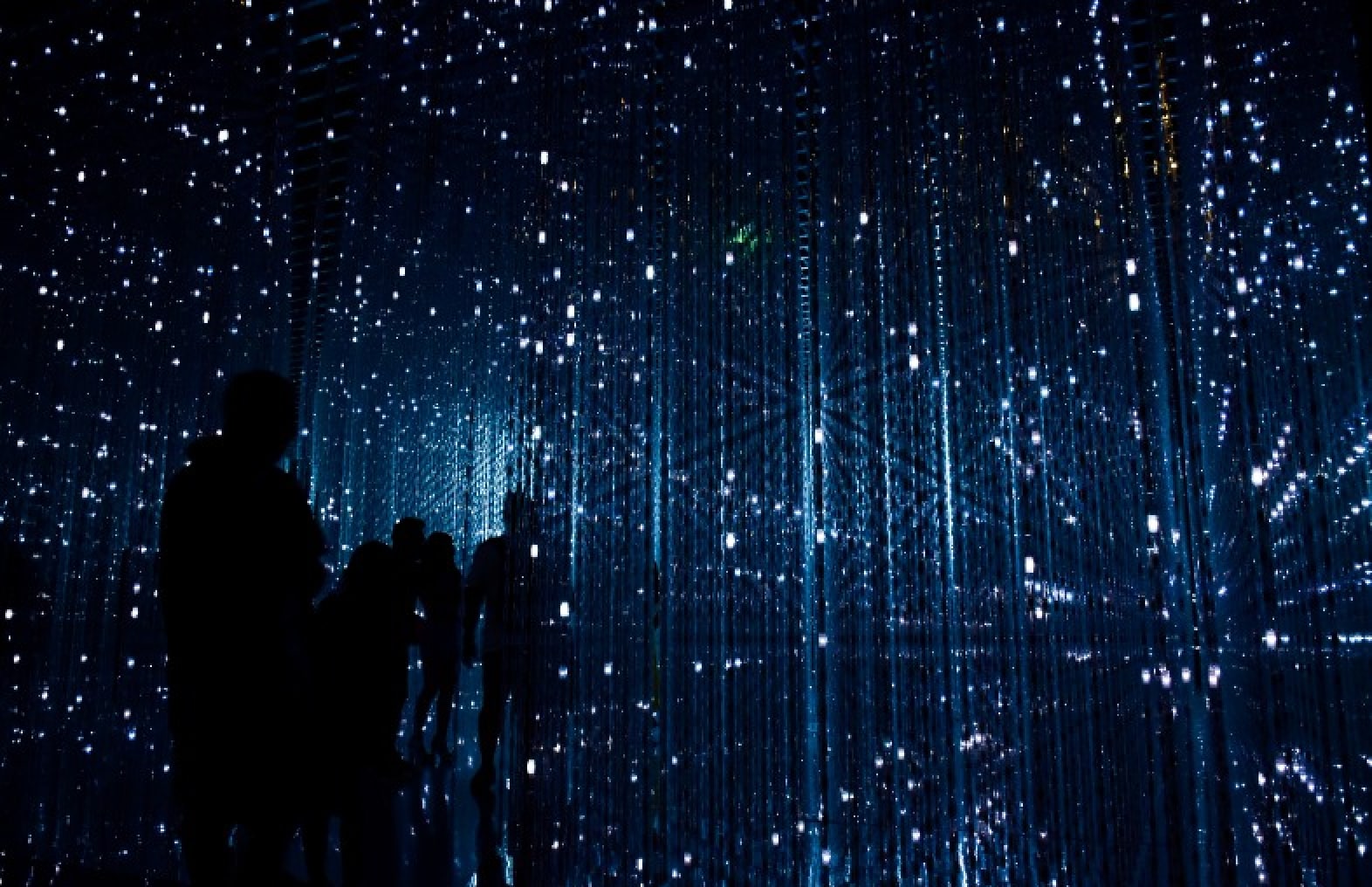
After the big announcement at JavaOne about JavaFX, I thought it’s time to give it a try. There is an Eclipse plugin available that helps creating JavaFX applications. Because I found some people having trouble getting the plugin to run, I wrote this small post to help out. You can use Eclipse’s update function to download the plugin from the following location.
http://download.java.net/general/openjfx/plugins/eclipse/site.xml
To get started you have to create a Java Project first. After doing this you can create JavaFX files from the “new” wizard.
This creates an empty JavaFX file. In the documentation at https://openjfx.dev.java.net/Getting_Started_With_JavaFX.html there are some nice examples to get started with. Add the following code to your JavaFX file for example:
import javafx.ui.*;
import java.lang.System;
class PhotoModel {
attribute currentPhoto : String;
attribute photos : String*;
attribute photoIndex : int;
operation nextPhoto();
operation previousPhoto();
}
operation PhotoModel.nextPhoto() {
if(photoIndex + 1 < sizeof photos) {
this.currentPhoto = photos[++this.photoIndex];
} else {
this.photoIndex = 0;
this.currentPhoto = photos[0];
}
}
operation PhotoModel.previousPhoto() {
if(photoIndex – 1 >= 0) {
this.currentPhoto = photos[–this.photoIndex];
} else {
this.photoIndex = (sizeof photos) – 1;
this.currentPhoto = photos[this.photoIndex];
}
}
var photoModel = new PhotoModel();
photoModel.photos = [“https://duke.dev.java.net/images/JDK6/DukeWithHelmet.png”,
“https://duke.dev.java.net/images/web/Duke_Blocks.gif”,
“https://duke.dev.java.net/images/china/DukeWithDragonSmall.jpg”,
“https://duke.dev.java.net/images/glassfish/GlassFishMedium.jpg”];
photoModel.currentPhoto = photoModel.photos[0];
Frame {
title: “JavaFX Demo!”
width: 600
height: 700
content:
BorderPanel {
border: EmptyBorder {
top: 30
left: 30
bottom: 50
right: 30
}
center:
Label {
text: bind “<html><body><img src='{photoModel.currentPhoto}’/></body></html>”
}
bottom:
FlowPanel {
content:
[Button {
text: “Previous”
action: operation() {
photoModel.previousPhoto();
}
},
RigidArea {
width: 5
},
Button {
text: “Next”
mnemonic: I
action: operation() {
photoModel.nextPhoto();
}
}]
}
}
background: black
visible: true
}
The only thing left to do is creating a run configuration. You’ll need to use ‘net.java.javafx.FXShell’ as the Main class, and put the name of the file you want to run as an argument of the application.
The code example above creates simple photoviewer. Not something you can’t do with Swing or HTML, but JavaFX makes it a lot easier. The result will look like this:
I must say that after the little time I played with JavaFX I really start to like it. It takes almost no time at all to create a simple UI that looks cool and has some basic functionality. I’ll be playing a lot more with it next week, and i’m hoping to show some more advanced examples soon. I’m also wondering about the possibilities to run JavaFX from a browser, but I’m sure we’ll see and hear more about this soon.
Some good places to start reading about JavaFX are Chris Oliver’s blog and the project’s page at dev.java.net.