Static Noise
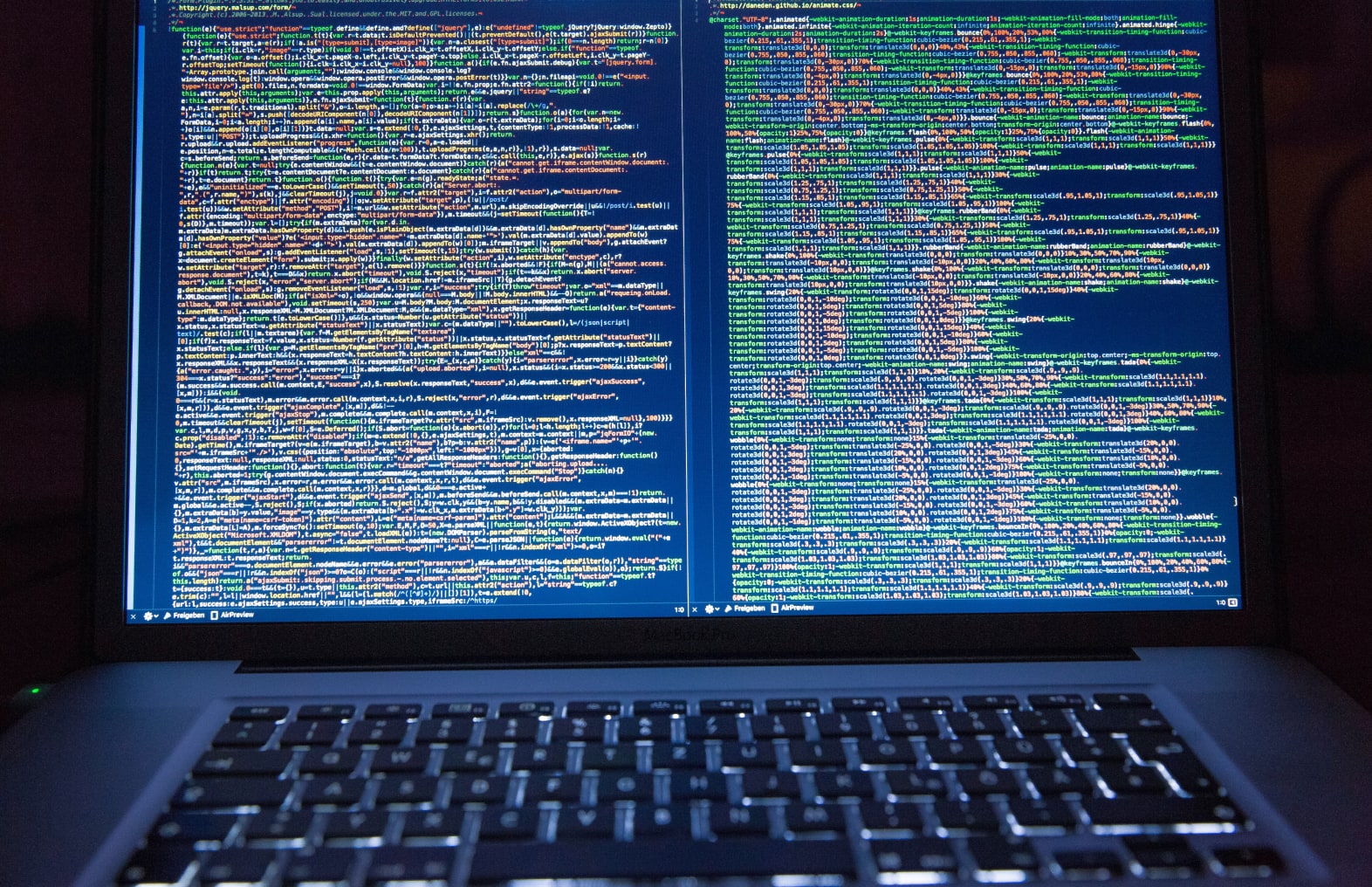
Today a question of a colleague put me back on one of my hobby horses: the danger of static. I have a true abhorrence for class members. For C#: static fields and properties, for Java static attributes and for Visual Basic .NET: Shared fields and properties. To me ‘static’ is one of those things that should have never been allowed in the (modern) object oriented languages. Let me explain my concerns.
Static means that the property is an attribute of the type. Unfortunately this is commonly explained as a shared value across all instances of the type. Even worse, starting programmers interpret this as: “I have to use static when a property of a class has the same value for all instances of that class”. Which is different because it illegally inverts the ‘rule’.
Let’s have a look at a classic example: a bank account class and its interest attribute. All accounts must have the same interest level and it would be pretty hard to implement this requirement by keeping the values synchronized across all instances. It would appear that this attribute is a good candidate to become a static property of the bank account class. Now suppose you implement the requirement by using a static property. All seems fine until that dark day comes when the program needs to be adjusted to accommodate not just the bank accounts of a single bank but of multiple banks. These banks do not share the same interest level. So what to do? Change the static to an instance property? Create a bank account class for each bank?
Every time I run into questions like these I know there is a flaw in my design. In this case I think that the assumption that the interest is an attribute of the bank accounts is wrong. I think that it is an attribute of the bank or an attribute of the association between bank and its bank accounts. Suddenly I can do without the static.
Another thing that has been bothering me for a long time is the static main. C#, Java and Visual Basic .NET have all inherited (pun intended!) this entry point from C. Which, in my humble opinion, is pure nonsense. Instead of explaining what is wrong with it, let me propose a more object oriented solution. As you may know a Java program is started (on the command line) by starting the virtual machine and passing it the name of the class that contains the static main. Like this: java MyFirstBank I propose a tiny adjustment: instead of having this line calling the static main of the class have it call the constructor of the class, optionally an overload of the constructor with fitting parameters. As much as this seems trivial it will take away the strange requirement of the static main and on top of that this will allow you to run any class as a program.
My final worry: many programmers that have a lot of experience with procedural programming in C or in VB6 or other, similar languages have trouble understanding object orientation. So two things happen: 1. The first thing they are confronted with is the static main, a (sort of) global function. You know, that evil thing we are trying to unlearn. 2. Static allows these starting OO programmers to fall back to old habits.
I think that in 99% of the cases we can do without static properties (if you use them make sure you use some kind of synchronization/locking scheme because you share the property across all threads!!)
In short: Static invites bad design.
If you feel like replying to this post with examples of code that can only be implemented by using static please do so AFTER you have tried to solve the problem without using statics 😉 For example: do not assume that you really need a static to create a singleton. First be creative and try to create a singleton without using static and after you have done that investigate your solution and try to find what you have learned about statics and singletons…